Impressione-se
Descubra como Python está presente em seu dia-a-dia.
Inicie-se
Veja como é fácil começar a usar a linguagem.
Aprenda mais
Conheça mais sobre a linguagem e torne-se um verdadeiro pythonista.
Participe
Encontre e participe da comunidade e compartilhe suas dúvidas e idéias.
APyB
Conheça a Associação Python Brasil.
Código de Conduta
Código de Conduta da comunidade Python Brasil.
Grupos de Usuários Python no Brasil (GUPy)
GruPy-ES
Grupo de usuários do Espírito Santo
GruPy-RP
Grupo de usuários de Ribeirão Preto
Python Sorocaba
Grupo de usuários de Sorocaba
Python Floripa
Grupo de usuários de Florianópolis
grupy-sanca
Grupo de usuários Python de São Carlos (SP)
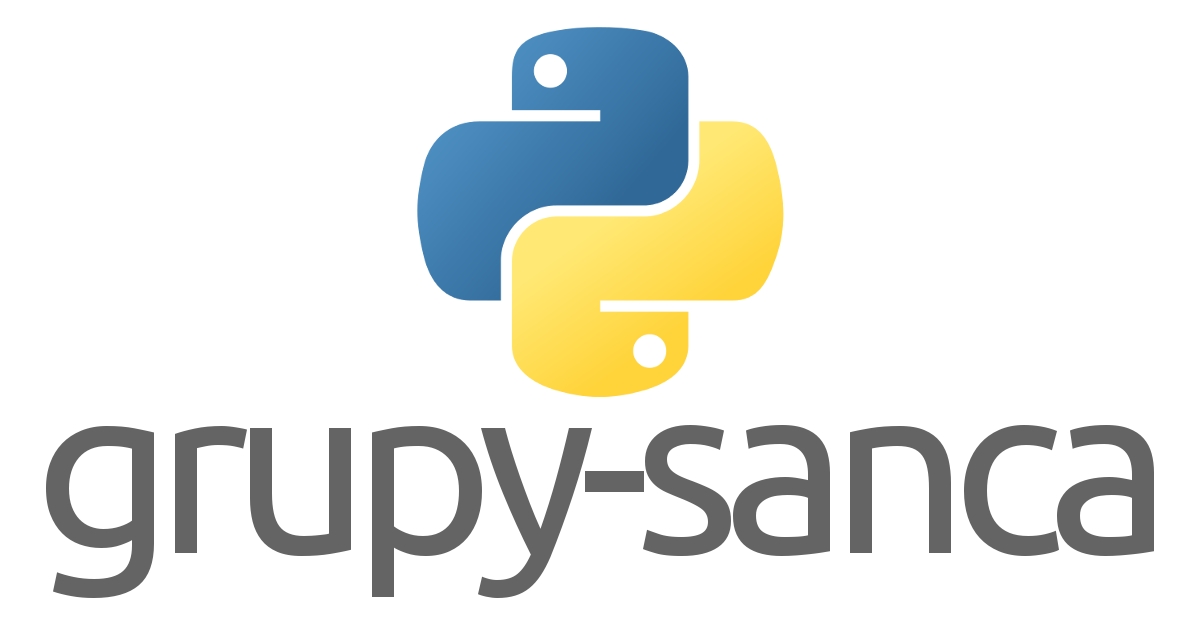
Pug-SC
Grupo de usuários de Santa Catarina
Py013
Grupo de usuários da Baixada Santista DDD 013 (Litoral de SP)
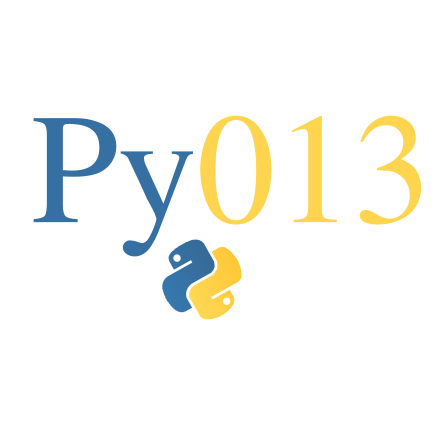